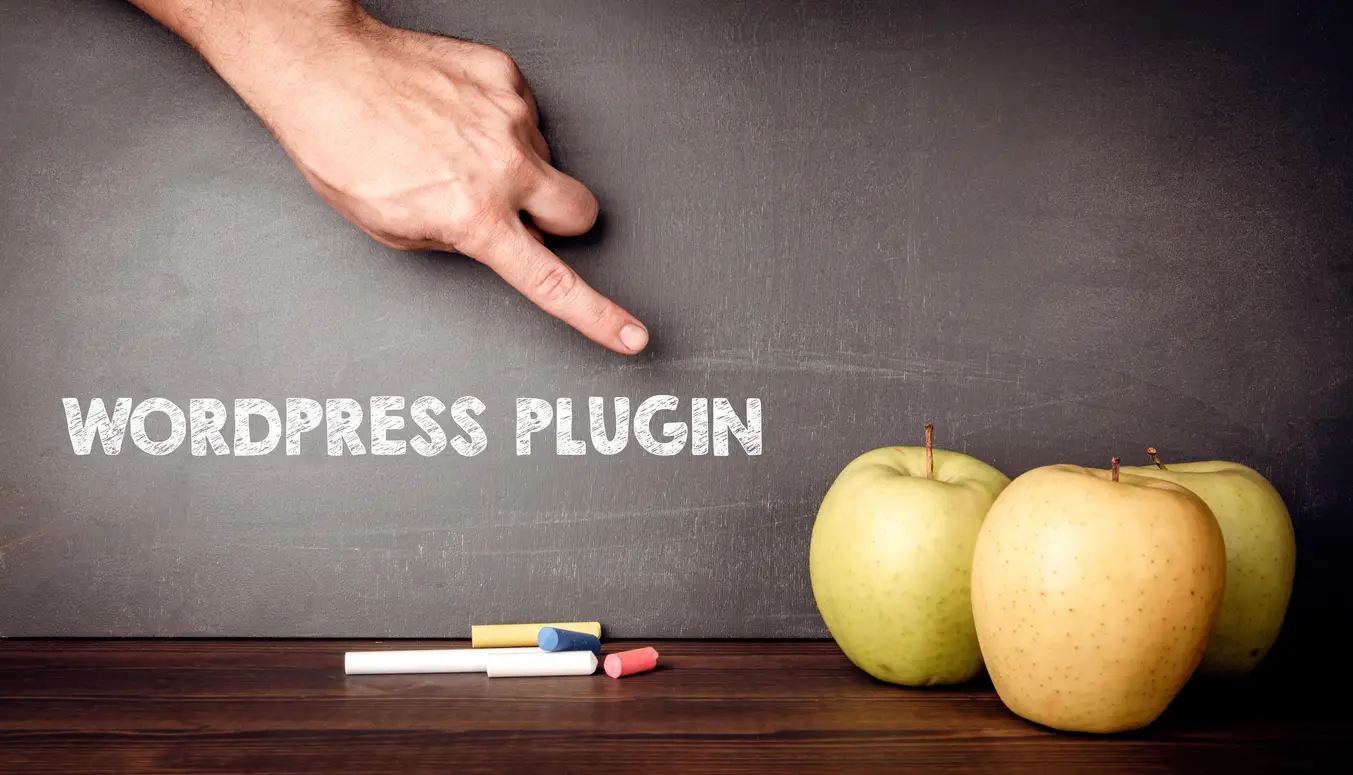
How to Create Your Own WordPress Plugins: From Concept to Deployment
WordPress powers over 40% of all websites on the internet, making it the most popular content management system (CMS) worldwide. One key factor contributing to its success is the ability to extend its functionality through plugins. Developing custom plugins for WordPress allows you to add new features, modify existing ones, and tailor the CMS to your specific needs. This comprehensive guide’ll walk you through creating your own WordPress plugins, from concept to deployment.
Understanding WordPress Plugin Architecture
Before diving into plugin development, it’s crucial to understand the basic architecture of WordPress plugins. A WordPress plugin is a collection of PHP files that interact with the WordPress core through a system of hooks and filters.
Key Components of WordPress Plugins:
- Plugin Header: A comment block at the top of the main plugin file that provides essential information about the plugin.
- Functions: Custom PHP functions that define the plugin’s functionality.
- Hooks: Actions and filters that allow your plugin to interact with WordPress core and other plugins.
- Shortcodes: Special tags that can add dynamic content to posts and pages.
- Widgets: Custom sidebar elements that users can add and customize.
Understanding these components will help you create well-structured and efficient plugins.
Setting Up Your Development Environment
To start developing custom plugins for WordPress, you’ll need a proper development environment. Here’s what you’ll need:
- Local WordPress Installation: Set up a local WordPress site using tools like XAMPP, MAMP, or Local by Flywheel.
- Code Editor: Choose a code editor or IDE that supports PHP development. Popular options include Visual Studio Code, Sublime Text, or PhpStorm.
- Version Control: Use Git for version control and consider hosting your code on platforms like GitHub or GitLab.
- Browser Developer Tools: Familiarize yourself with browser developer tools for debugging and testing.
Once your environment is set up, you can start planning your custom plugin.
Planning Your Custom Plugin
Before writing any code, it’s important to define your plugin’s purpose and functionality clearly. Consider the following:
- Plugin Objective: What problem does your plugin solve, or what functionality does it add?
- Target Audience: Who will use your plugin?
- Required Features: List the specific features your plugin needs to include.
- WordPress Integration: Determine how your plugin will interact with WordPress core and other plugins.
- User Interface: Plan any admin pages, settings, or front-end elements your plugin will need.
Taking the time to plan your plugin thoroughly will save you time and effort during the development process.
Writing the Plugin Code
Now that you have a plan, it’s time to start coding your custom WordPress plugin. Here’s a basic structure to get you started:
- Create a new folder in your WordPress plugins directory (
wp-content/plugins/
). - Create the main plugin file (e.g.,
my-custom-plugin.php
). - Add the plugin header comment to the main file:
<?php
/*
Plugin Name: My Custom Plugin
Plugin URI: https://example.com/my-custom-plugin
Description: A brief description of your plugin
Version: 1.0
Author: Your Name
Author URI: https://example.com
License: GPL2
*/
- Start adding your plugin’s functionality using WordPress hooks and functions. Here’s a simple example:
function my_custom_plugin_init() {
// Plugin initialization code
}
add_action('init', 'my_custom_plugin_init');
function my_custom_plugin_shortcode($atts) {
// Shortcode functionality
return 'Hello from My Custom Plugin!';
}
add_shortcode('my_custom_shortcode', 'my_custom_plugin_shortcode');
- Organize your code into separate files for better maintainability:
includes/
: For helper functions and classesadmin/
: For admin-related functionalitypublic/
: For front-end functionalityassets/
: For CSS, JavaScript, and images
Remember to use WordPress coding standards and best practices while developing your plugin.
Testing and Debugging
Thorough testing is crucial for developing reliable WordPress plugins. Here are some testing strategies:
- Manual Testing: Test your plugin’s functionality on different WordPress versions and with various themes.
- Automated Testing: Use PHPUnit for unit testing your plugin’s functions.
- Cross-browser Testing: Ensure your plugin works correctly across different web browsers.
- Performance Testing: Check that your plugin doesn’t negatively impact site performance.
- Security Testing: Use tools like OWASP ZAP to identify potential security vulnerabilities.
For debugging, use WordPress’s built-in debugging features by adding the following to your wp-config.php
file:
define('WP_DEBUG', true);
define('WP_DEBUG_LOG', true);
define('WP_DEBUG_DISPLAY', false);
This will log errors to a debug.log file in the wp-content directory.
## Deploying Your Custom Plugin
Once your plugin is tested and ready, you can deploy it for use on live WordPress sites. Here are the steps:
1. **Prepare Your Plugin**: Ensure all files are properly organized and commented.
2. **Create a README file**: Include installation instructions, usage guide, and any other relevant information.
3. **Version Control**: If you're using Git, create a release tag for your plugin version.
4. **Distribution**: You can distribute your plugin through the WordPress Plugin Directory or as a premium plugin through your own website or marketplaces.
To submit your plugin to the WordPress Plugin Directory:
1. Create an account on WordPress.org
2. Submit your plugin for review
3. Once approved, upload your plugin files using SVN
## Best Practices for WordPress Plugin Development
To ensure your custom WordPress plugins are high-quality and well-received, follow these best practices:
1. **Follow WordPress Coding Standards**: Adhere to the [WordPress Coding Standards](https://developer.wordpress.org/coding-standards/wordpress-coding-standards/) for consistent and readable code.
2. **Use Prefixes**: Prefix your function names, classes, and global variables to avoid conflicts with other plugins or themes.
3. **Implement Security Measures**: Sanitize and validate user input, use nonces for form submissions, and follow WordPress security best practices.
4. **Optimize Performance**: Minimize database queries, use transients for caching, and enqueue scripts and styles properly.
5. **Internationalization**: Make your plugin translatable by using WordPress internationalization functions.
6. **Regular Updates**: Maintain your plugin by fixing bugs, adding new features, and ensuring compatibility with new WordPress versions.
7. **Documentation**: Provide clear documentation for users and developers who might work with your plugin.
8. **Modular Design**: Design your plugin with modularity in mind, making it easier to maintain and extend in the future.
## Conclusion
Developing custom plugins for WordPress is a powerful way to extend the functionality of the world's most popular CMS. By following the steps outlined in this guide and adhering to best practices, you can create high-quality plugins that enhance WordPress sites and provide value to users.
Remember that plugin development is an ongoing process. Continuously learn from user feedback, stay updated with WordPress developments, and refine your skills to create even better plugins in the future.
Are you ready to start developing your custom WordPress plugin? Share your ideas or experiences in the comments below, and don't forget to subscribe to our newsletter for more WordPress development tips and tutorials!
[Learn more about WordPress plugin development](https://developer.wordpress.org/plugins/)